Web Design II
Class 04: JavaScript PracticeTopics
- Introduction to JQuery
- Intermediate JavaScript Examples
- Class 04: Single Page Site (jquery components) Lab
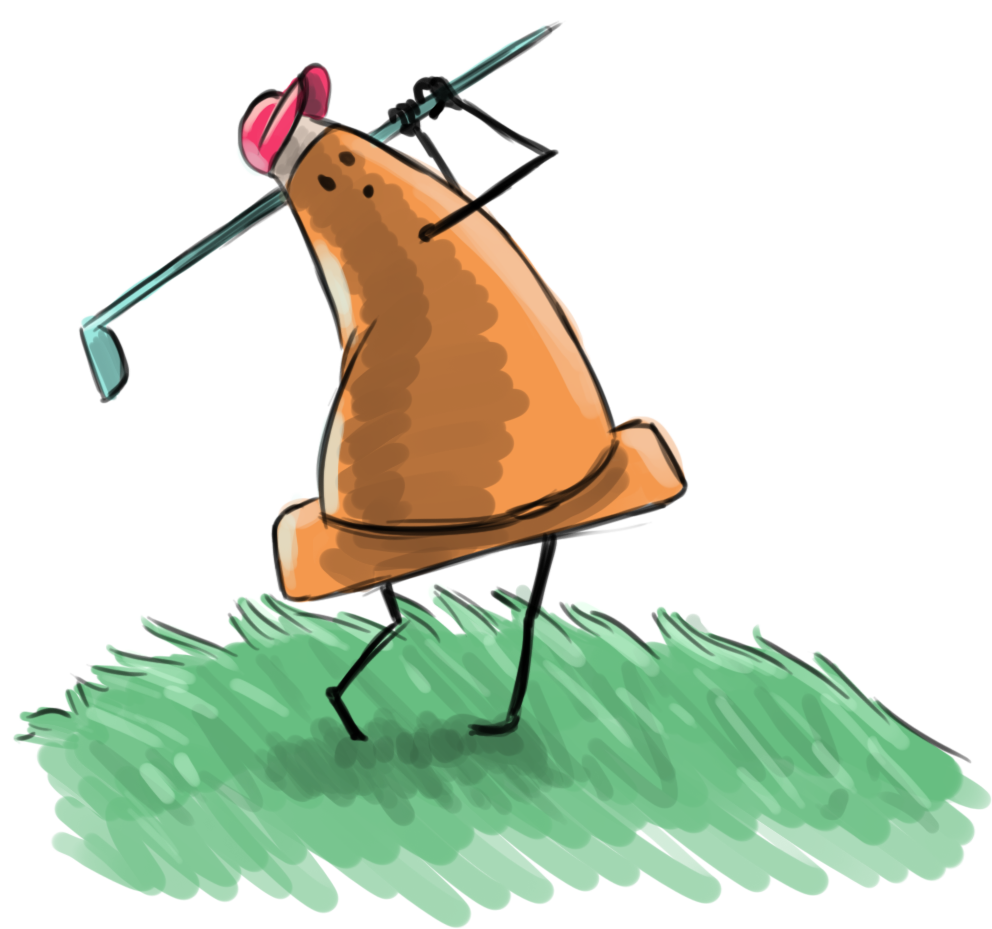
I hope you enjoy class Fore!
Introduction to JQuery
JQuery:
JQuery is a library of JavaScript. It basically abbreviates JavaScript code into smaller, simpler lines. This is very similar to using BootStrap (in fact JQuery is one of the components of BootStrap).
Attaching jQuery:
There are two primary ways to incorporate jQuery into your site.
-
- Host it yourself:
- You can download the code and link it to your site to use much like an external CSS sheet.
- You can download it here.
- <script src=”js/jquery-3.4.1.min.js”></script>
- CDN (Content Delivery Network):
- Instead of downloading and hosting the code yourself you can simply link it from a popular source.
- <script src=”https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js”></script>
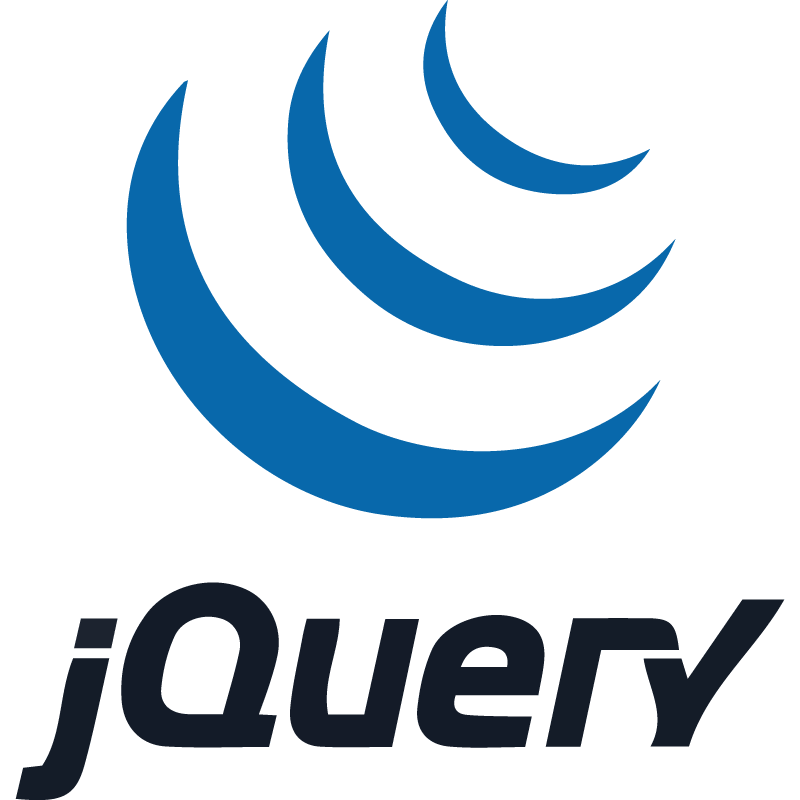
Syntax:
The structure of jQuery is actually pretty straight-forward.
- $
- Denotes jQuery usage.
- (selector)
- The element the code is attached to.
- .action()
- The thing that happens.
Ready:
All jQuery code should generally be placed inside a ready function. This makes sure that webpage is fully loaded before the jQuery runs.
- $(document).ready(function(){
- jQuery code here
- });
jQuery Examples:
Here are some quick jQuery snippets.
- Hide and Show
- Used to quickly hide or show an element
- Example
- Toggle
- Single method that works like a switch to turn on or off visibility.
- Example
- Fade
- Sets elements to appear via opacity over a period of time.
- Example
- Slide
- Like an accordion, makes elements visible and expand into view.
- Example
- Animate
- Much like CSS you can animate elements but with much more control.
- Example
More jQuery:
There are many more things jQuery can do.
To see more information on jQuery visit here.
Intermediate JavaScript Examples
Responsive Navbar Tutorial Video
Responsive Navbar:
Navigation that collapses to a single menu button when shrunk and expands vertically when clicked.
HTML:
- <ul id=”navbar”>
- Encompass the entire unordered list menu bar.
- <li class=”nav” id=”menuBtn”><a href=”#” onclick=”openMenu()”>
- The list item that holds the link that when clicked runs the openMenu function.
- <div id=”hamburger”><p>-</p><p>-</p><p>-</p></div>
- Simply three “-” meant to represent the menu icon.
- <li class=”nav”><a href=”#”>Home</a></li>
- Each list item that has the links attached have the nav class to target them.
CSS:
- body {}
- font-family:Arial, Helvetica, Sans-serif; margin:0px; box-sizing:border-box;
- Style the general default content.
- a:link, a:visited {}
- color:rgb(255, 255, 255); background-color:rgb(131, 131, 131); display:inline-block; width:100px; padding:10px; text-align:center; text-decoration:none; font-weight:700;
- Style the links to look nicer.
- a:hover {}
- color:rgb(0, 0, 0); background-color:rgb(194, 194, 194);
- Style the color of the links when the mouse is over them.
- #menuBtn {}
- display:none;
- Turn off the menu button by default.
- li {}
- list-style-type:none; display:inline;
- Have the list (links) items stack horizontally by default.
- #hamburger p {}
- position:relative; font-size:2em; transform:scale(4, 1); margin:0px; padding:0px; top:0px; line-height:10px;
- Create a menu button by stack p elements and formatting them.
- @media screen and (max-width: 600px) {}
- li {list-style-type:none;}
- #menuBtn {display:block;}
- .nav{display:none;}
- Turn off the horizontal links and turn on the menu button.
- @media screen and (max-width: 600px) {}
- .resMenu {display:block;}
- Turn on the responsive vertically stacked menu.
JavaScript:
- function openMenu() {
- Function that will run when the button is pressed.
-
- var navEl = document.getElementsByClassName(“nav”);
- Variable that stores the nav elements.
- var i;
- Variable that stores the counter.
- for (i = 1; i < navEl.length; i++) {
- Starting at 1 (not 0), and for the number of nav elements, run the following code.
-
- if (navEl[i].className === “nav”){
- If the current nav element has the nav class attached.
-
- navEl[i].className += ” resMenu”;}
- Add the resMenu class to it as well.
- else {
- Else do this.
-
- navEl[i].className = “nav”;}
- Set the attached class to just nav.
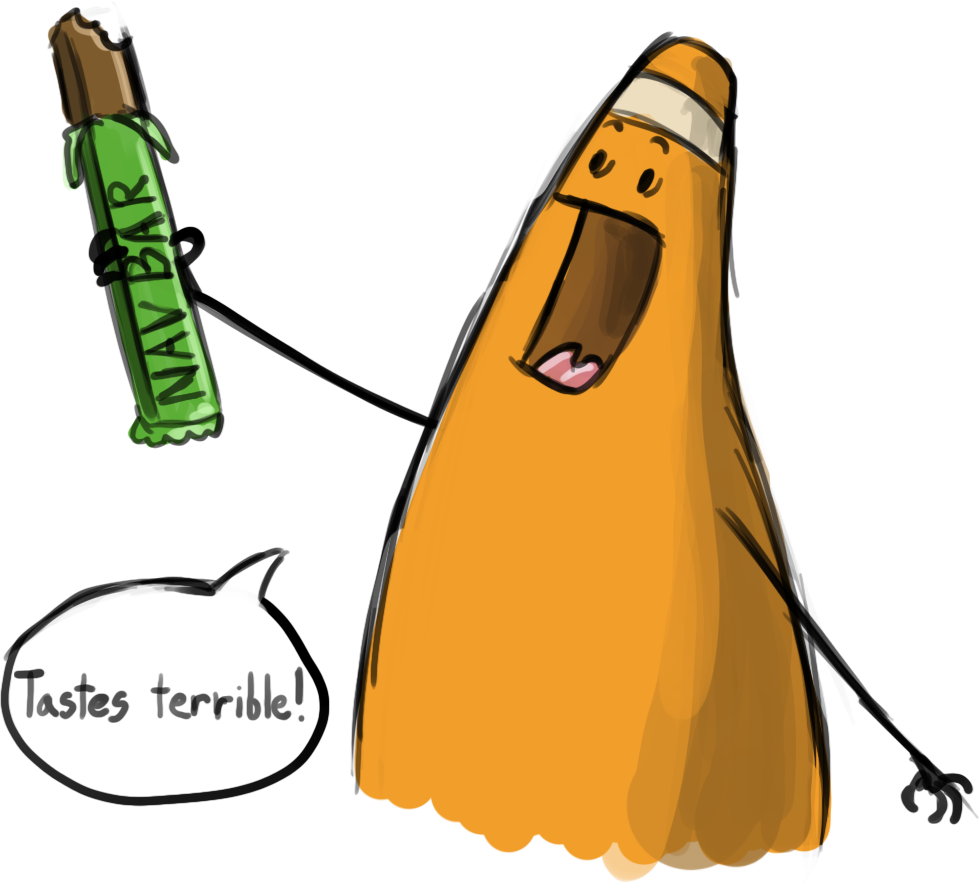
Navbar now comes in responsive. “Tastes terrible!”
Responsive Navbar HTML
Responsive Navbar CSS
#hamburger p {
Responsive Navbar JavaScript
Slider Tutorial Video
Slider (carousel, slideshow, etc.):
Create a list of images that the user can click through.
HTML:
- <div class=”slideshow”>
- Div that holds the entire slider
- <div class=”slide”>
- Div for each slide. Repeat the same code for more slides.
- <img src=”images/photo_1.png” class=”slideImg” />
- The actual image attached.
- <div class=”caption”>
- Div to hold caption for each image slide.
- <a class=”prev” onclick=”nextSlide(-1)>❮</a>
- The previous button that runs the nextSlide function when pressed.
- <a class=”next” onclick=”nextSlide(1)>❯</a>
- The next button that runs the nextSlide function when pressed.
- <div id=”slideIndicator”>
- Holds the “dots” that represent each slide.
- <span class=”dot” onclick=”currentSlide(1)”></span>
- Each dot is a link to that particular image slide.
CSS:
- * {}
- box-sizing:border-box;
- Make sure borders and padding to not extend past width on all elements.
- body {}
- font-family:Verdana, Sans-serif; margin:0;
- Set the default font and margin for the page.
- .slide {}
- display:none;
- By default turn off all slides.
- .slideImg {}
- vertical-align:middle; width:100%;
- Set the slide images to span the entire page and be aligned in the center vertically.
- .slideshow {}
- max-width:1000px; position:relative; margin:auto;
- Makes sure the slideshow does not get too big and is centered in the page.
- .prev, .next {}
- cursor:pointer; position:absolute; top:50%; width:auto; padding:16px; margin-top:-22px; color:white; font-weight:bold; transition:0.6s ease; border-radius: 0 3px 3px 0; user-select:none;
- Generally formatting the next and previous buttons.
- .next {}
- right:0px; border-radius:3px 0 0 3px;
- Format the next button so it sticks to the right side of the slider.
- .prev:hover, .next:hover {}
- background-color:rgba(0, 0, 0, 0.8);
- Style the previous and next buttons when the mouse is over it.
- .caption {}
- color: #f2f2f2; font-size:15px; padding:8px 12px; position:absolute; bottom:8px; width:100%; text-align:center;
- Format the caption section.
- #slideIndicator {}
- text-align:center;
- Center the dots.
- .dot {}
- cursor:pointer; height:15px; width:15px; margin:0px; background-color:#bbb; border-radius: 50%; display:inline-block; transition: background-color 0.6s ease;
- Style the dot slide indicators.
- .active, .dot:hover {}
- background-color:#717171;
- Format the dots when the mouse is over them.
JavaScript:
- var slideNum = 1;
- Set the current slide number to 1;
- showSlide(slideNum);
- Run the showSlide function and send it the current slide number.
- function nextSlide(n) {
- Run the following code when called.
-
- showSlide(slideNum += n);}
- Run the showSlide function and send it the slideNum + n.
- function currentSlide(n) {
- Run the following code when called.
-
- showSlide(slideNum = n);}
- Run the showSlide function and send it the slideNum + n.
- function showSlide(n) {
- Run the following code when called.
-
- var i;
- Counter variable.
- var slides = document.getElementsByClassName(“slide”);
- Store all the elements with the “slide” class into the slides variable.
- var dots = document.getElementsByClassName(“dot”);
- Stores all the elements with the “dots” class into the dots variable.
- if (n > slides.length) {
- If n is less than the number of elements in the slides variable.
-
- slideNum = 1;}
- Set the value of slideNum to 1.
- if (n < 1) {
- if n is less than one.
-
- slideNum = slides.length;}
- Set the value of SlideNum to the total number of elements in the slides variable.
- for (i= 0; i < slides.length; i++) {
- Starting at 0 and run the following code the total number of elements in slides.
-
- slides[i].style.display = “none”;}
- Set the current slides element off.
- for (i = 0; i < dots.length; i++) {
- Starting at 0 and run the following code the total number of elements in dots.
-
- dots[i].className = dots[i].className.replace(” active”, “”);}
- Replace the class attached to dots with active.
- slides[slideNum – 1].style.display = “block”;
- Turn on the the slide.
- dots[slideNum – 1].className += ” active”;}
- Make the correct dot active.
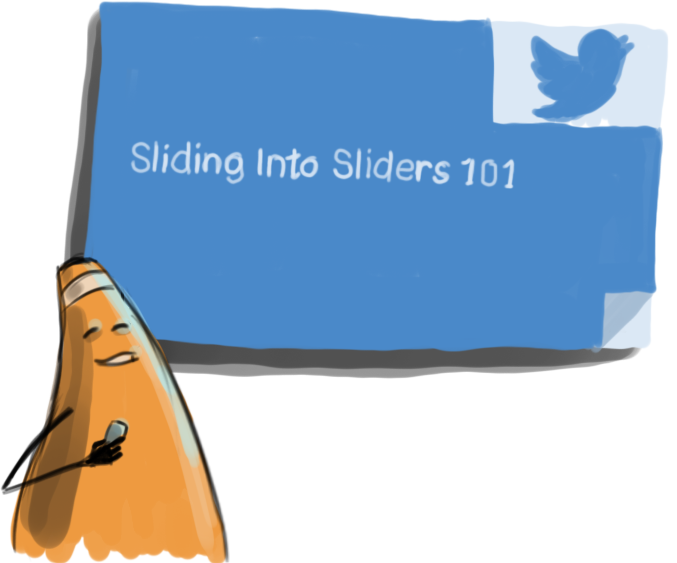
Slider HTML
Slider CSS
.slideshow {
.prev, .next {
.next {
.prev:hover, .next:hover {
.caption {
#slideIndicator {
.dot {
.active, .dot:hover {
Slider JavaScript
Class 04: Single Page Site (jquery components) Lab
Single Page Site (jquery components) Lab
n the last assignment you incorporated some simple JavaScript into your webpage. In this assignment you will once again incorporate JavaScript into your webpage but it will be slightly more complicated. You can choose to either develop a responsive navbar or slider.
You will be graded on the following:
- Lab Requirements
- Techniques and processes covered in the instructional material is followed and implemented.
- Creativity & Craftsmanship
- Excellent design choices, novel & appealing, and solid clean caliber work.
Resources:
- Assignment Video Tutorials
- You may watch these tutorial videos below to help you complete your assignment.
- Assignment Lab Materials
- You may use what you produced int he previous lab.
Lab Tutorial Slideshow
This tutorial will add a navbar and slider into our responsive webpage.
Wait! Before you go!
Did you remember to?
- Read through this webpage
- Watch the videos
- Submit Class 04: Single Page Site (jquery components) Lab on Blackboard
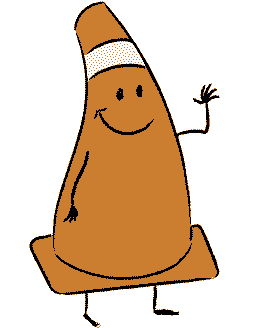