Web Design II
Class 02: Responsive CodeTopics
- Manual Responsive Code
- Class 02 Single Page Site (responsive) Lab
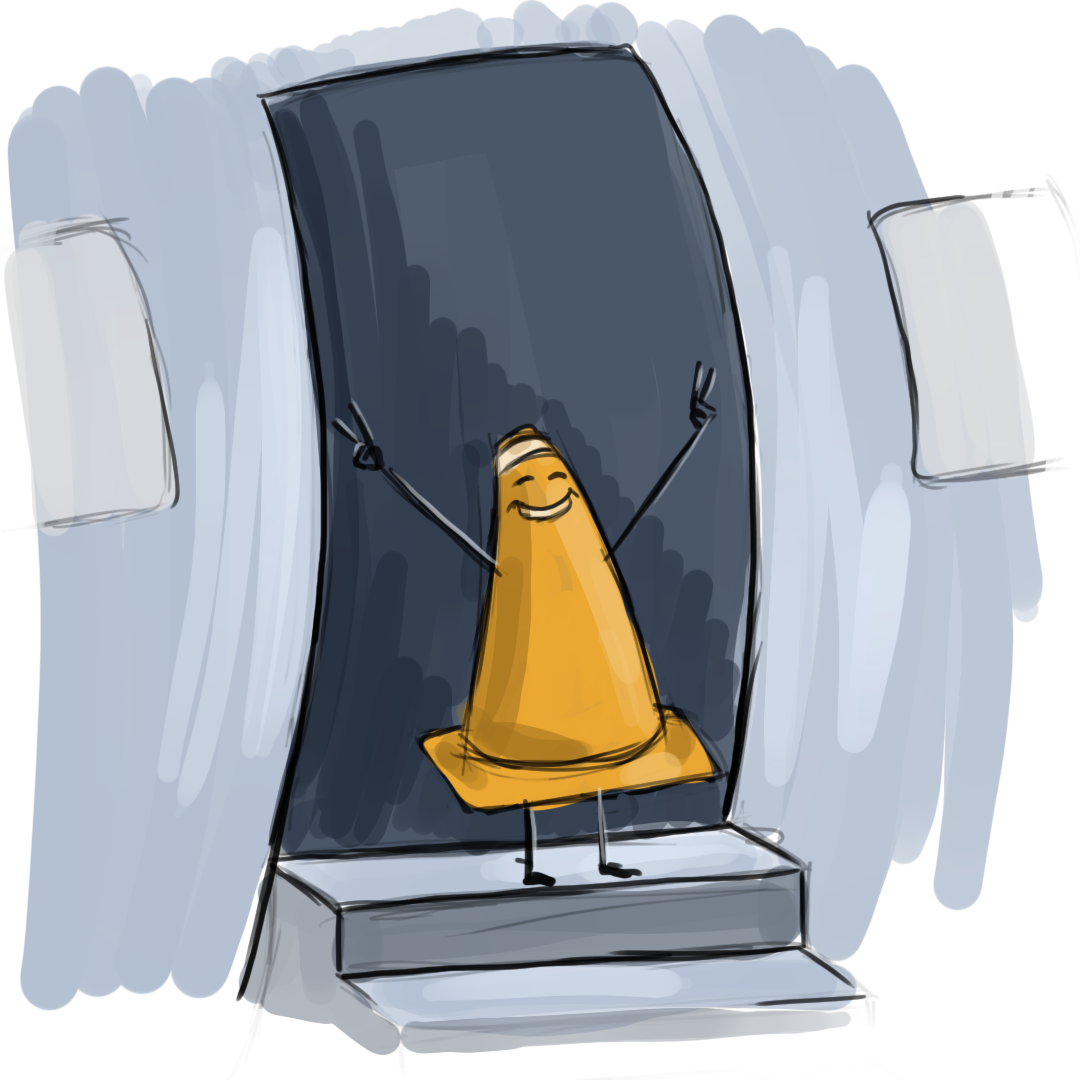
Welcome to class two!
Manual Responsive Code
Responsive Design:
Originally web pages were designed for computer screens specifically using a fixed-width static design. As tablets and phones have become more common to browse the internet those layouts do not transfer well. Enter responsive web design. A responsive layout will automatically change it’s styling to match the device it is viewed on.
Bootstrap:
Bootstrap is a framework written in CSS and JavaScript that web designers may use to incorporate a responsive grid into their web pages. There are many responsive templates out there but Bootstrap has become the standard given its popularity and ease of use.
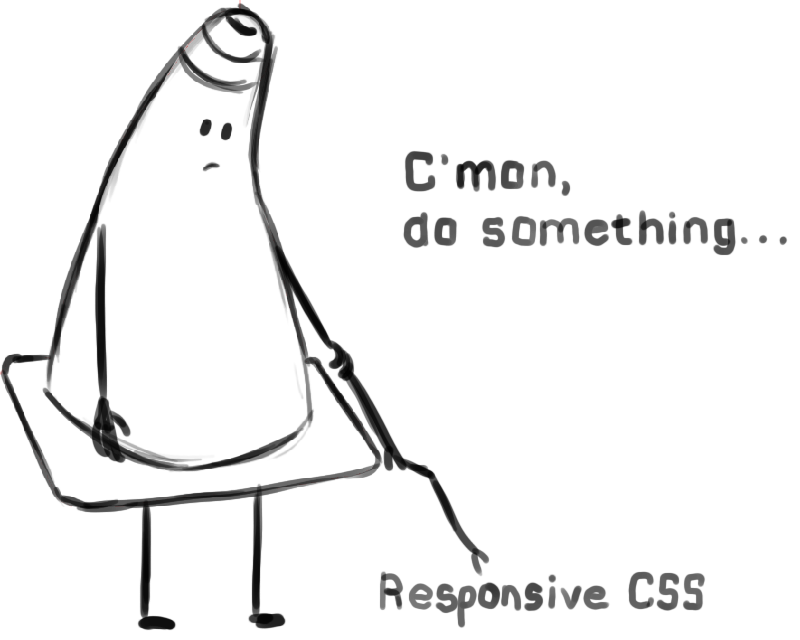
Basic Responsive Code Lecture
Basic Responsive Code:
Responsive tag:
This sized the viewport to the device dimensions and resets the zoom value.
- <meta>
- Viewport element that gives browser instructions.
- width=device-width
- Sets the page with to the width of the device.
- initial-scale=1.0
- Resets the scale value of zoom.
Column CSS:
like Bootstrap we will define 12 column classes.
- .col-1 { }
- You need to make 12 column classes (.col-1, .col-2, .col-3, etc.).
- width: 8.33%;
- To have 12 equal columns you take 100% divided by 12 and get 8.33%. Add that to each subsequent column for different sizes. For example, 8.33% * 8 = 66.66% for an column 8 and 8.33% * 5 = 41.66% for column 5.
- float: left;
- The columns should float to the left.
Row CSS:
Also like Bootstrap we need to create rows that will clear at the end so that a new row may start on a new line.
- .row::after { }
- Create a row class that selects the area after it using the ::after pseudo selector.
- content: “”;
- Inserts basically nothing into the space.
- clear: both;
- Doesn’t allow elements to on the left and right of the space.
- display: table;
- Allows the element to behave like a table element.
Other CSS
This CSS should be added so everything fits appropriately.
- box-sizing: border-box
- Attempts to fit elements’ height and width to the actual dimensions despite padding and border. Padding and border may add on top of the box dimensions causing them to be larger than what you wrote.
this is the title
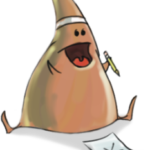
Hello There!
I’m ridiculously subtitle
This is so much content I can’t stand it
I am another subtitle
How does he do it? It’s some sort of black magic for sure!
Variable Column Sizing Lecture
Variable Column Sizing:
Media Query:
The media query will allow you to create CSS rules based on browser screen dimensions.
- @media
- Used to query the media type (size of the device).
- only
- Keyword that prevents older browsers that don’t support @media from from reading the subsequent CSS. There is also a not keyword that does the opposite of what the media query does as well as and that combines multiple features.
- screen
- Applying to the actual screen.
- (max-width: 600px)
- Size of window.
Column CSS:
We need column settings for different dimension (phone, tablet, desktop/laptop). Remove the old column classes (.col-1 … col-12) and replace with these:
- Extra Small Screen (less than 600px)
- The default sizing should be full width for the small screen.
- .col-1-s, .col-2-s, .col-3-s, .col-4-s, .col-5-s, .col-6-s, .col-7-s, .col-8-s, .col-9-s, .col-10-s, .col-11-s, .col-12-s {width: 100%;}
- Small Screen (greater than 600px)
- @media only screen and (min-width: 600px)
- { .col-1-s {width: 8.33%;} .col-2-s {width: 16.66%;} .col-3-s {width: 25%;} .col-4-s {width: 33.33%;} .col-5-s {width: 41.66%;} .col-6-s {width: 50%;} .col-7-s {width: 58.33%;} .col-8-s {width: 66.66%;} .col-9-s {width: 75%;} .col-10-s {width: 83.33%;} .col-11-s {width: 91.66%;} .col-12-s {width: 100%;} }
- Medium Screen (greater than 768px)
- @media only screen and (min-width: 768px)
- { .col-1-m {width: 8.33%;} .col-2-m {width: 16.66%;} .col-3-m {width: 25%;} .col-4-m {width: 33.33%;} .col-5-m {width: 41.66%;} .col-6-m {width: 50%;} .col-7-m {width: 58.33%;} .col-8-m {width: 66.66%;} .col-9-m {width: 75%;} .col-10-m {width: 83.33%;} .col-11-m {width: 91.66%;} .col-12-m {width: 100%;} }
- Larger Screen (greater than 992px)
- @media only screen and (min-width: 992px)
- { .col-1-l {width: 8.33%;} .col-2-l {width: 16.66%;} .col-3-l {width: 25%;} .col-4-l {width: 33.33%;} .col-5-l {width: 41.66%;} .col-6-l {width: 50%;} .col-7-l {width: 58.33%;} .col-8-l {width: 66.66%;} .col-9-l {width: 75%;} .col-10-l {width: 83.33%;} .col-11-l {width: 91.66%;} .col-12-l {width: 100%;} }
- Other CSS
-
- [class*=”col-“] {float: left;}
- This applies “float:left” to all classes that start with “col-“.
Other CSS:
Keep the rest of the CSS
- .row::after {content: “”; clear: both; display: table;}
- Keep the row clearing CSS rule.
- box-sizing: border-box
- Keep the the box-sizing border box rule so the elements fit properly.
this is the title
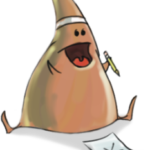
Hello There!
I’m ridiculously subtitle
This is so much content I can’t stand it
I am another subtitle
How does he do it? It’s some sort of black magic for sure!
this is the title
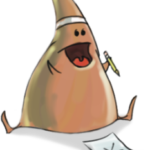
Hello There!
I’m ridiculously subtitle
This is so much content I can’t stand it
I am another subtitle
How does he do it? It’s some sort of black magic for sure!
Responsive Elements:
Responsive Text:
You may want to adjust text size depending on the screen size.
- @media only screen and (max-width:600px){}
- Tests against screen size and apply CSS if the condition is true.
- .responsive-h {}
- Make whatever class name you’d like to target what elements it applies to.
- font-size: .5em;
- Adjusts the size of the type.
Responsive Images:
You may want the image to adjust size based on the screen so it is not too large or small.
- .responsive-img {}
- This could be anything but you need to create a class selector to affect only the images you want.
- max-width: 100%;
- This will scale the image according the containing element but not beyond its source resolution.
- height: auto;
- Will automatically scale the height proportionally to the width.
Responsive Background Image:
If using a background image you will probably want it to scale to the screen size.
- body {}
- Typically you apply the background image to the body if you want it to span the entire page.
- width: 100%;
- Making the width span across the screen.
- height: auto;
- Set the height to match the width proportionally.
- background-image: url(‘images/backgroundImage.png’);
- Attach an image.
- background-size: cover;
- This will allow the background to scale but will crop as opposed to stretching the image.
- background-repeat: no-repeat;
- You may want to have the background repeat but most of the time it isn’t advisable.
Hide Elements:
You may want certain elements to only be visible at certain resolutions. In this example I have a detailed logo for a large screen and a basic thumbnail logo for a smaller screen.
- @media only screen and (max-width: 600px)
- Keep the row clearing CSS rule.
- .logoLarge {}
- Create a selector to target the larger logo element.
- display: none;
- Make the element disappear.
- @media only screen and (min-width: 600px)
- Keep the row clearing CSS rule.
- .logoSmall {}
- Create a selector to target the smaller logo element.
- display: none;
- Make the element disappear.
this is the title
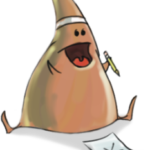
Hello There!
I’m ridiculously subtitle
This is so much content I can’t stand it
I am another subtitle
How does he do it? It’s some sort of black magic for sure!
this is the title
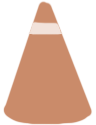
Hello There!
I’m ridiculously subtitle
This is so much content I can’t stand it
I am another subtitle
How does he do it? It’s some sort of black magic for sure!
Class 02 Single Page Site (responsive) Lab
Single Page Site (responsive) Lab:
In web design I you learned to develop a responsive website utilizing Bootstrap. Although this is the most commonly used framework and you should continue to use it, there is power in the ability to create your own custom responsive CSS. In this lab you will convert the previous fixed-width document into a responsive one by writing your own CSS. We may also take the opportunity to rework the page and add more elements.
You will be graded on the following:
- Basic Document & File Structure:
- Master folder created with correct sub folders.
- All files named and placed correctly.
- Basic HTML and CSS structure produced.
- Responsive Column Layout:
- Sections are broken down into rows and columns
- At least three levels of columns are produced (sm, md, lg)
- Responsive Elements:
- Images are responsive
- Text is responsive
- Aesthetics:
- Interesting and appealing design.
- Descriptive and well-written text
Resources:
- Assignment Video Tutorials
- You may watch these tutorial videos below to help you complete your assignment.
- Assignment Lab Materials
- You may download the images used in lab here: webWeek01LabMaterials.
Lab Tutorial Slideshow
This tutorial will review the HTML and CSS that was covered in Web Design I.
Wait! Before you go!
Did you remember to?
- Read through this webpage
- Watch the videos
- Submit Class 02 Single Page Site (responsive) Lab on Blackboard
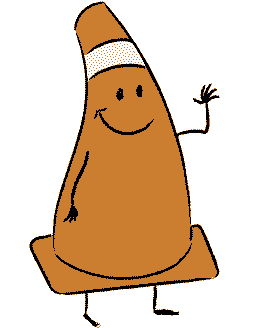